jq + 面向对象实现拼图游戏
知识点
- 拖拽事件
- es6面向对象
- jquery事件
- 效果图
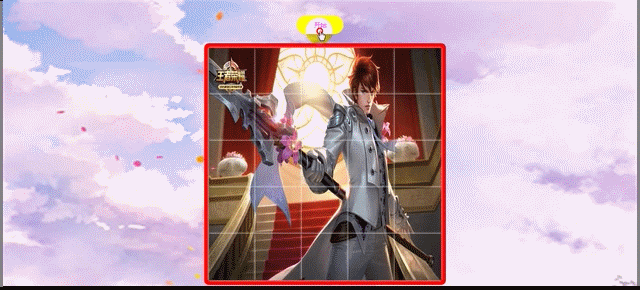
html:
<div class="wraper">
<div class="btn">
<button class="start">开始</button>
</div>
<div class="box"></div>
</div>
css:
* {
margin: 0;
padding: 0;
list-style: none;
}
html,
body {
width: 100%;
height: 100%;
background: url('../img/bg_pic.jpg') no-repeat;
background-size: 100% 100%;
display: flex;
justify-content: center;
align-items: center;
flex-direction: column;
}
.wraper {
width: 500px;
height: 600px;
position: relative;
}
.wraper .btn {
text-align: center;
line-height: 80px;
}
.wraper .btn button {
width: 100px;
height: 40px;
background: yellow;
border: none;
outline: none;
font-size: 14px;
color: red;
border-radius: 20px;
cursor: pointer;
}
.wraper .box {
width: 100%;
height: 500px;
position: relative;
border: 10px solid red;
border-radius: 10px;
}
.wraper .box .pic {
position: absolute;
background: url('../img/zy.jpg') no-repeat;
box-shadow: 0 0 5px #fff;
background-size: 500px 500px;
cursor: pointer;
}
js:
class Game {
constructor() {
this.boxW = parseInt($('.box').css('width'));
this.boxH = parseInt($('.box').css('height'));
this.imgW = this.boxW / 5;
this.imgH = this.boxH / 5;
this.flag = true; //true为开始 false为重排
this.orArr = []; //标准数组
this.randArr = []; //乱序数组
this.init();
}
init() {
this.createDom();
this.getState();
}
createDom() {
//行
for (var i = 0; i < 5; i++) {
//列
for (var j = 0; j < 5; j++) {
this.orArr.push(i * 5 + j);
let imgs = $("<div class='pic'></div>").css({
width: this.imgW + 'px',
height: this.imgH + 'px',
left: j * this.imgW + 'px',
top: i * this.imgH + 'px',
backgroundPosition: -j * this.imgW + 'px ' + -i * this.imgH + 'px'
});
$('.box').append(imgs);
}
}
}
getState() {
let btn = $('.btn .start');
let imgs = $('.pic');
let _this = this;
btn.on('click', function() {
if (_this.flag) {
_this.flag = false;
btn.text('重排');
_this.getRandom();
_this.getOrder(_this.randArr);
imgs.on('mousedown', function(e) {
let index = $(this).index();
let left = e.pageX - imgs.eq(index).offset().left;
let top = e.pageY - imgs.eq(index).offset().top;
$(document).on('mousemove', function(e1) {
let left1 = e1.pageX - left - $('.box').offset().left - 10;
let top1 = e1.pageY - top - $('.box').offset().top - 10;
imgs.eq(index).css({
'z-index': '40',
'left': left1,
'top': top1
})
}).on('mouseup', function(e2) {
let left2 = e2.pageX - left - $('.box').offset().left - 10;
let top2 = e2.pageY - top - $('.box').offset().top - 10;
let index2 = _this.changeIndex(left2, top2, index);
if (index === index2) {
_this.picReturn(index);
} else {
_this.picChange(index, index2);
}
$(document).off('mousemove').off('mouseup').off('mousedown');
})
return false;
})
} else {
_this.flag = true;
btn.text('开始');
_this.getOrder(_this.orArr);
imgs.off('mousemove').off('mouseup').off('mousedown');
}
})
}
changeIndex(left, top, index) {
if (left < 0 || left > this.boxW || top < 0 || top > this.boxH) {
return index;
} else {
let col = Math.floor(left / this.imgW);
let row = Math.floor(top / this.imgH);
let moveIndex = 5 * row + col;
let i = 0;
let len = this.randArr.length;
while ((i < len) && this.randArr[i] !== moveIndex) {
i++;
}
return i;
}
}
picReturn(index) {
let j = this.randArr[index] % 5;
let i = Math.floor(this.randArr[index] / 5);
$('.pic').eq(index).css('z-index', '40').animate({
'left': j * this.imgW,
'top': i * this.imgH
}, 300, function() {
$(this).css('z-index', '10');
})
}
picChange(index, index2) {
let _this = this;
let fromJ = _this.randArr[index] % 5;
let fromI = Math.floor(_this.randArr[index] / 5);
let toJ = _this.randArr[index2] % 5;
let toI = Math.floor(_this.randArr[index2] / 5);
let temp = _this.randArr[index];
$('.pic').eq(index).css('z-index', '40').animate({
'left': toJ * _this.imgW + 'px',
'top': toI * _this.imgH + 'px'
}, 300, function() {
$(this).css('z-index', '10');
})
$('.pic').eq(index2).css('z-index', '40').animate({
'left': fromJ * _this.imgW + 'px',
'top': fromI * _this.imgH + 'px'
}, 300, function() {
$(this).css('z-index', '10');
_this.randArr[index] = _this.randArr[index2];
_this.randArr[index2] = temp;
_this.check();
})
}
getRandom() {
this.randArr = [...this.orArr];
this.randArr.sort(function() {
return Math.random() - 0.5;
})
}
getOrder(arr) {
let len = arr.length;
for (var i = 0; i < len; i++) {
$('.box .pic').eq(i).animate({
left: arr[i] % 5 * this.imgW,
top: Math.floor(arr[i] / 5) * this.imgH
}, 400)
}
}
check() { //判断是否成功
if (this.randArr.toString() == this.orArr.toString()) {
alert('拼图成功');
this.flag = true;
$('.btn .start').text('开始');
$('.pic').off('mousemove').off('mouseup').off('mousedown');
}
}
}
new Game();
参考至腾讯课堂渡一教育